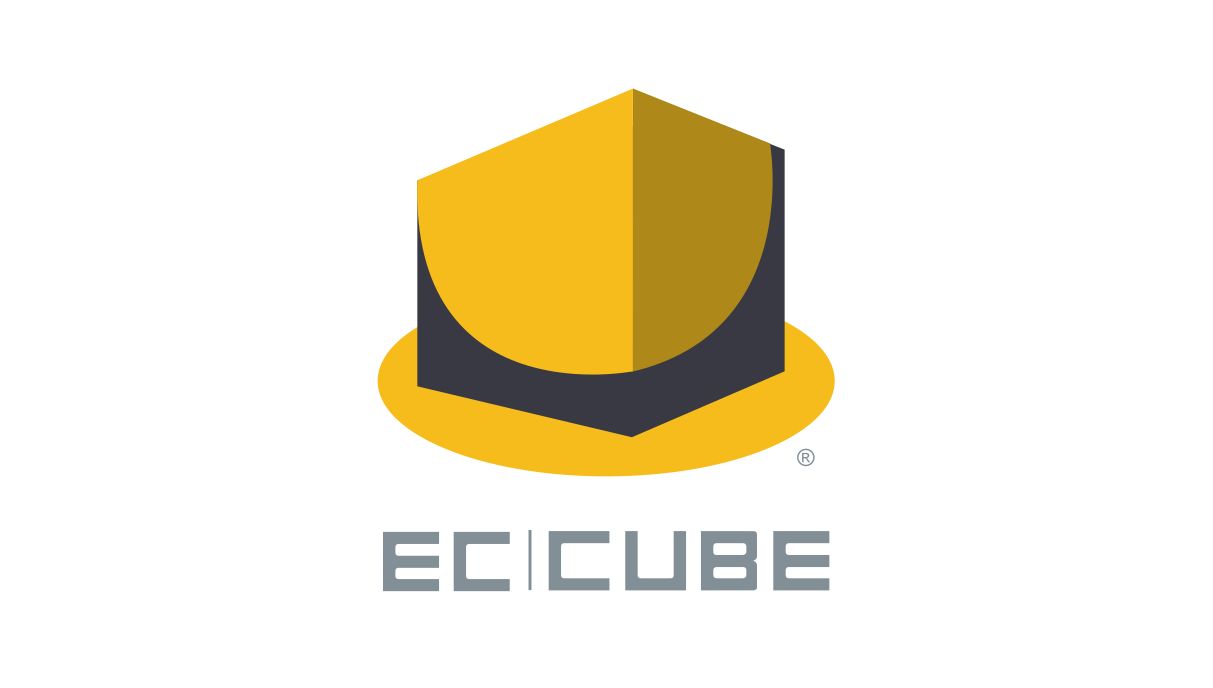
【EC-CUBE4】新規テーブル&カラム追加方法
こんにちは、みよしやです。
今回は、EC-CUBE4.1を使用して、新規テーブル作成と既存テーブルへのカラム追加方法を書いていきます。
既存テーブルへのカラム追加方法
カラムを追加するには、対象のEntityクラスを拡張するtraitという機能を使用します。
まずは、traitの定義方法と使用方法をご紹介します。
// traitの定義(変数や関数の書き方はclassの場合と変わりません)
trait SampleTrait {
// 処理
}
// traitの使用(useで読み込むことで、SampleTrait内の関数を使用することができます。
class SampleClass {
use [パス]\SampleTrait;
}
[traitの定義・使用例]
traitの作成
では実際にtraitの作成を行っていきます。
会員テーブル(dtb_customer)にFAX番号カラム(fax_number)を追加する例で説明します。
app\Customize\EntityにCustomerTrait.phpを作成します。
<?php namespace Customize\Entity; use Doctrine\ORM\Mapping as ORM; use Eccube\Annotation\EntityExtension; /** * @EntityExtension("Eccube\Entity\Customer") */ trait CustomerTrait { /** * @var string|null * * @ORM\Column(name="fax_number", type="string", length=14, nullable=true) */ private $fax_number; /** * Set fax_number. * * @param string|null $fax_number * * @return CustomerTrait */ public function setFaxNumber($fax_number = null) { $this->fax_number = $fax_number; return $this; } /** * Get fax_number. * * @return string|null */ public function getFaxNumber() { return $this->fax_number; } }
変数やgetter,setterの定義方法はEntityクラスと同一のため、
拡張元のクラス(今回はCustomer.php)を参考にしてみてください。
proxyファイルの生成
php [EC-CUBEインストールディレクトリ]/bin/console eccube:generate:proxies
traitの作成が完了したら、コマンドでproxyファイルの生成を行います。
class Customer extends \Eccube\Entity\AbstractEntity implements UserInterface, \Serializable
{
use \Customize\Entity\CustomerTrait;
上記のように、先ほど作成したCustomerTraitがuseで読み込まれていれば成功です。
DB反映コマンドの実行
// キャッシュクリア
php [EC-CUBEインストールディレクトリ]/bin/console cache:clear --no-warmup
// テーブル追加SQL実行('--force'を付けずに叩くと、実行予定のSQL文が表示されます)
php [EC-CUBEインストールディレクトリ]/bin/console eccube:schema:update --dump-sql --force
最後に、上記コマンドでDBに反映させたらカラム追加完了となります。
新規テーブルの作成方法
今度は新規のテーブルを作成する方法をご紹介します。
テーブル作成には、EntityとRepositoryが必要となります。
今回はdtb_sampleというテーブルを作成してみます。
Entityの作成
<?php
/*
* This file is part of EC-CUBE
*
* Copyright(c) EC-CUBE CO.,LTD. All Rights Reserved.
*
* http://www.ec-cube.co.jp/
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
namespace Customize\Entity;
use Doctrine\ORM\Mapping as ORM;
if (!class_exists('\Customize\Entity\Sample')) {
/**
* Sample
*
* @ORM\Table(name="dtb_sample")
* @ORM\InheritanceType("SINGLE_TABLE")
* @ORM\DiscriminatorColumn(name="discriminator_type", type="string", length=255)
* @ORM\HasLifecycleCallbacks()
* @ORM\Entity(repositoryClass="Customize\Repository\SampleRepository")
*/
class Sample extends \Eccube\Entity\AbstractEntity
{
/**
* @var int
*
* @ORM\Column(name="sample_column01", type="integer", options={"unsigned":true})
* @ORM\Id
* @ORM\GeneratedValue(strategy="NONE")
*/
private $sample_column01;
/**
* @var int
*
* @ORM\Column(name="sample_column02", type="integer", options={"unsigned":true})
* @ORM\Id
* @ORM\GeneratedValue(strategy="NONE")
*/
private $sample_column02;
/**
* Set sampleColumn01.
*
* @param int $sampleColumn01
*
* @return Sample
*/
public function setSampleColumn01($sampleColumn01)
{
$this->sample_column01 = $sampleColumn01;
return $this;
}
/**
* Get sampleColumn01.
*
* @return int
*/
public function getSampleColumn01()
{
return $this->sample_column01;
}
/**
* Set sampleColumn02.
*
* @param int $sampleColumn02
*
* @return Sample
*/
public function setSampleColumn02($sampleColumn02)
{
$this->sample_column02 = $sampleColumn02;
return $this;
}
/**
* Get sampleColumn02.
*
* @return int
*/
public function getSampleColumn02()
{
return $this->sample_column02;
}
}
}
まずはapp\Customize\EntityにSample.phpを追加します。
Repositoryの作成
<?php
/*
* This file is part of EC-CUBE
*
* Copyright(c) EC-CUBE CO.,LTD. All Rights Reserved.
*
* http://www.ec-cube.co.jp/
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
namespace Customize\Repository;
use Customize\Entity\Sample;
use Eccube\Repository\AbstractRepository;
use Symfony\Bridge\Doctrine\RegistryInterface;
/**
* SampleRepository
*
* This class was generated by the Doctrine ORM. Add your own custom
* repository methods below.
*/
class SampleRepository extends AbstractRepository
{
public function __construct(RegistryInterface $registry)
{
parent::__construct($registry, Sample::class);
}
}
次にapp\Customize\RepositoryにSampleRepository.phpを追加します。
DB反映コマンドの実行
// キャッシュクリア
php [EC-CUBEインストールディレクトリ]/bin/console cache:clear --no-warmup
// テーブル追加SQL実行('--force'を付けずに叩くと、実行予定のSQL文が表示されます)
php [EC-CUBEインストールディレクトリ]/bin/console eccube:schema:update --dump-sql --force
そしてカラム追加の時と同様に、上記コマンドを実行するとDBに反映されます。
最後に
EC-CUBEをカスタマイズする際、既存テーブルだけでは実装が難しい場合がありましたら、
今回の記事を参考に、独自のテーブルやカラムの追加を検討してみてはいかがでしょうか。
ご覧いただきありがとうございました。